Today i finally prepared test environment for SAP JSON proxy and i’d like to present all of you some of ideas.
First – why this project?
Right now i’m working on few applications which works with SAP but all of them are .net apps and fat client. Because i’m last time fascinated web and mobile programming again i was wondering how to easy build simple applications interacting with SAP. Usually it was quite easy – i was building web service which was exchanging data with my application. This was good idea and everything was working really nice and smooth, but problem was that first i have to do something on server and then in my app (web or mobile). Then i asked myself what if i will simply put universal application on web which will somehow understand some queries from my app and then will execute my calls on SAP and return data back.
I was wondering about exchanging data in xml but json seams to be better so i decided to use json.
How it works?
application is sending json object with definition of process which want to call on SAP, then proxy analyze this object and prepare RFC call for SAP. After call is executed and return is converted to json and send back to application.
What are benefit so this approach?
First of all – you don’t need to care about any special sap connectors for web and mobile devices. Second you don’t need to care about server side any more, you have to focus only on side of your application. Third it is secure because applications doesn’t have any direct access to SAP server so you can extend proxy by adding some security filters or limitations on calls
So now an example
Example works on bank accounts settings. On SAP we have BAPI_BANK_GETLIST bapi which looks this way:
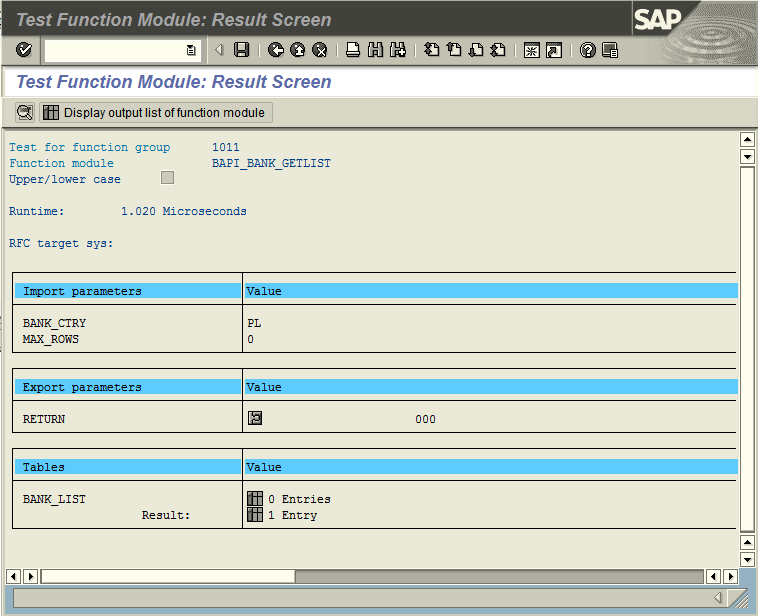
it expect import parameter BANK_CTRY – which in our example will be PL and returns BANK_LIST table which in our example looks this way:
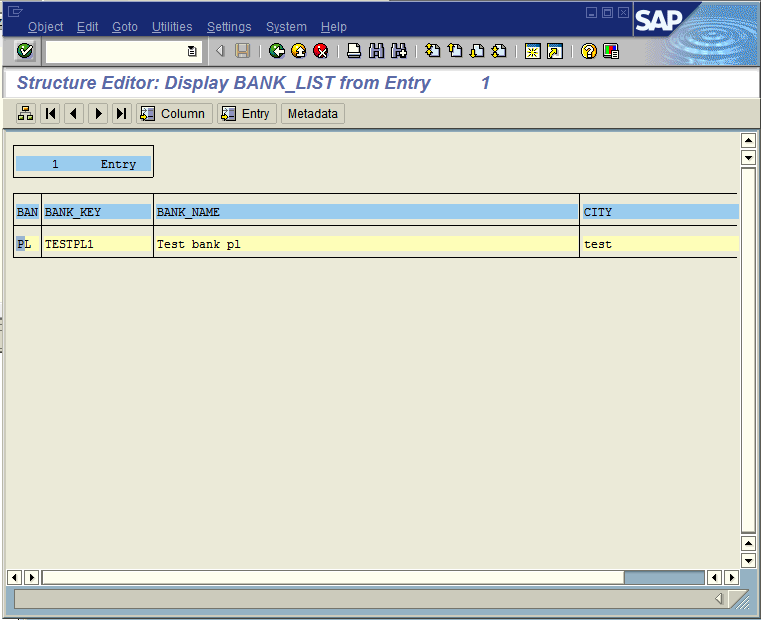
As you can see there is one row with one example bank.
my JSON query looks this way:
{ "bapiName" : "BAPI_BANK_GETLIST",
"errorMessage" : "",
"exportFields" : [ { "name" : "BANK_CTRY",
"value" : "PL"
},
{ "name" : "MAX_ROWS",
"value" : "0"
}
],
"exportStructures" : [ ],
"importFields" : [ ],
"importStructures" : [ { "fields" : [ { "name" : "TYPE",
"value" : " "
},
{ "name" : "ID",
"value" : " "
},
{ "name" : "NUMBER",
"value" : "000"
},
{ "name" : "MESSAGE",
"value" : " "
},
{ "name" : "LOG_NO",
"value" : " "
},
{ "name" : "LOG_MSG_NO",
"value" : "000000"
},
{ "name" : "MESSAGE_V1",
"value" : " "
},
{ "name" : "MESSAGE_V2",
"value" : " "
},
{ "name" : "MESSAGE_V3",
"value" : " "
},
{ "name" : "MESSAGE_V4",
"value" : " "
},
{ "name" : "PARAMETER",
"value" : " "
},
{ "name" : "ROW",
"value" : "0"
},
{ "name" : "FIELD",
"value" : " "
},
{ "name" : "SYSTEM",
"value" : " "
}
],
"name" : "RETURN"
} ],
"result" : "OK",
"tables" : [ { "columns" : [ "BANK_CTRY",
"BANK_KEY",
"BANK_NAME",
"CITY"
],
"name" : "BANK_LIST",
"rows" : [ ]
} ]
}
and proxy response is this:
{ "bapiName" : "BAPI_BANK_GETLIST",
"errorMessage" : "",
"exportFields" : [ { "name" : "BANK_CTRY",
"value" : "PL"
},
{ "name" : "MAX_ROWS",
"value" : "0"
}
],
"exportStructures" : [ ],
"importFields" : [ ],
"importStructures" : [ { "fields" : [ { "name" : "TYPE",
"value" : ""
},
{ "name" : "ID",
"value" : ""
},
{ "name" : "NUMBER",
"value" : "000"
},
{ "name" : "MESSAGE",
"value" : ""
},
{ "name" : "LOG_NO",
"value" : ""
},
{ "name" : "LOG_MSG_NO",
"value" : "000000"
},
{ "name" : "MESSAGE_V1",
"value" : ""
},
{ "name" : "MESSAGE_V2",
"value" : ""
},
{ "name" : "MESSAGE_V3",
"value" : ""
},
{ "name" : "MESSAGE_V4",
"value" : ""
},
{ "name" : "PARAMETER",
"value" : ""
},
{ "name" : "ROW",
"value" : "0"
},
{ "name" : "FIELD",
"value" : ""
},
{ "name" : "SYSTEM",
"value" : ""
}
],
"name" : "RETURN"
} ],
"result" : "OK",
"tables" : [ { "columns" : [ "BANK_CTRY",
"BANK_KEY",
"BANK_NAME",
"CITY"
],
"name" : "BANK_LIST",
"rows" : [ { "values" : [ { "name" : "BANK_CTRY",
"value" : "PL"
},
{ "name" : "BANK_KEY",
"value" : "TESTPL1"
},
{ "name" : "BANK_NAME",
"value" : "Test bank pl"
},
{ "name" : "CITY",
"value" : "test"
}
] } ]
} ]
}
And this is how it looks in my HTML tester page which works similar to SE37 transaction
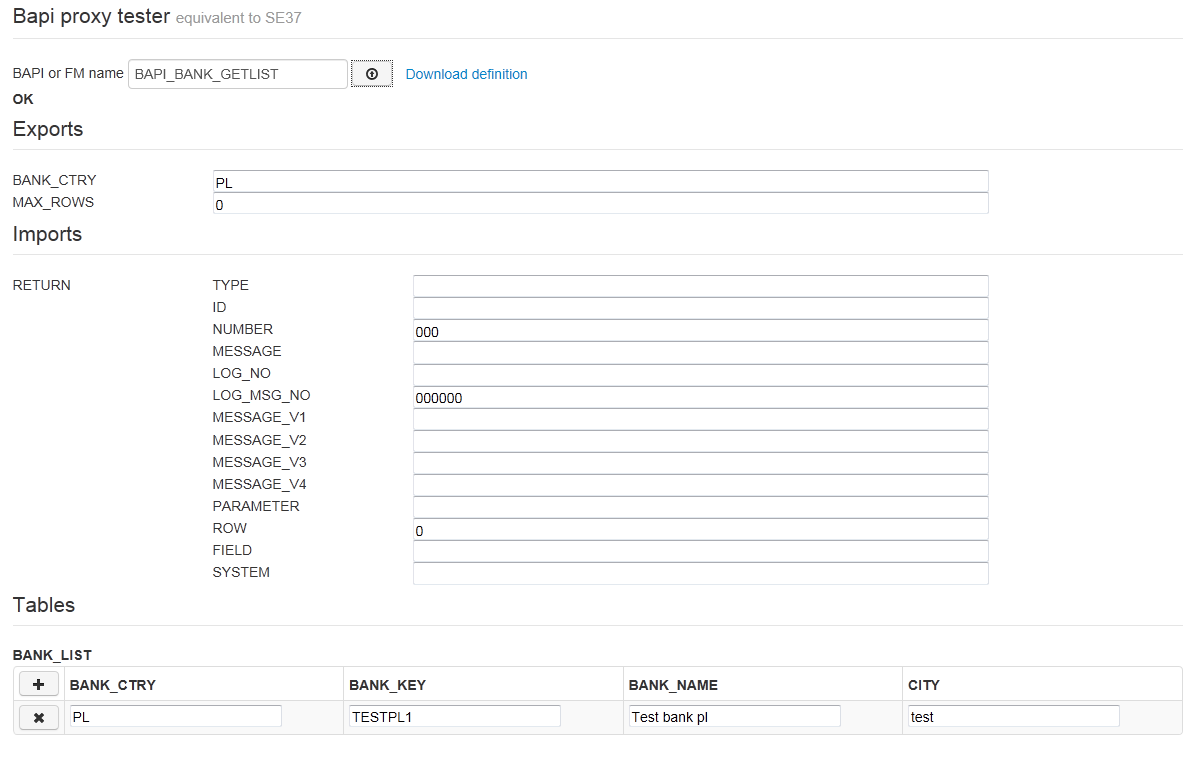
And here is another example:
Details of this bank account:
And my data
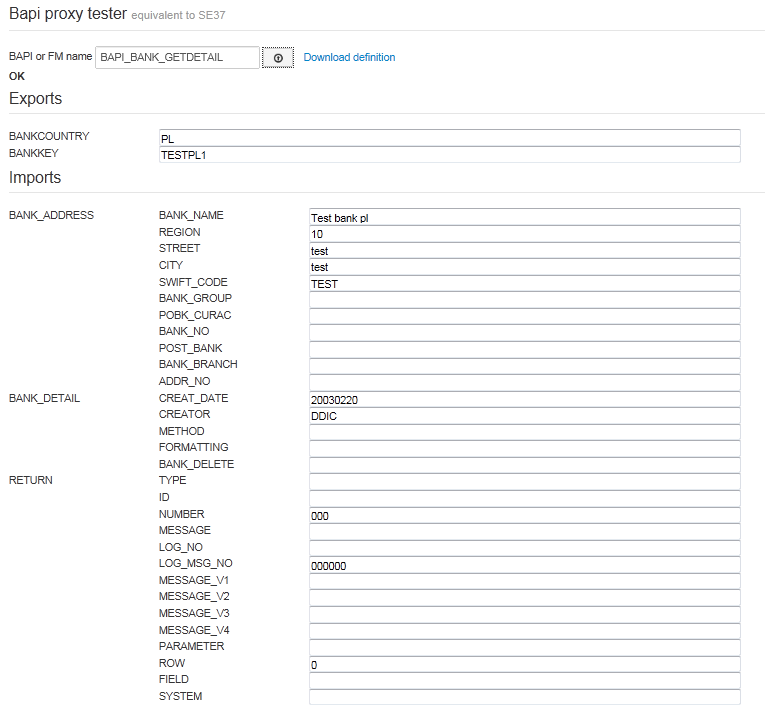
This is current stage, if you have any questions for this project, then please let me know in comments or send an email.